8. Install the MongoDB driver for Node.js. Create a Node.js script to connect to the shop database. Implement insert, find, update, and delete operations using the Node.js MongoDB driver. Define a product schema using Mongoose. Insert data into the products collection using Mongoose. Create an Express API with a /products endpoint to fetch all products. Use fetch in React to call the /products endpoint and display the list of products. Add a POST /products endpoint in Express to insert a new product. Update the Product List, After adding a product, update the list of products displayed in React.
Step 1: Create Project:
- Create a folder and open with vscode.
- Open integrated vscode terminal.
Step 2: Set up the Backend:
- Run the following commands to set up the environment for your project:
- Run one by one command to install the mongoose.
mkdir shop-backend
cd shop-backend
Step 3: Initialize Node.js Project:
npm init -y
Step 4: Install Dependencies:
npm install express mongoose mongodb cors
Step 5: Create server.js file:
- Right click on shop-backend folder and create the server.js file.
- Copy the below code and paste it in that file, save it.
const express = require('express');
const mongoose = require('mongoose');
const cors = require('cors');
const app = express();
app.use(cors());
app.use(express.json());
// MongoDB Connection
mongoose.connect('mongodb://localhost:27017/shop', {
useNewUrlParser: true,
useUnifiedTopology: true
});
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', () => console.log('Connected to MongoDB'));
// Define Mongoose Schema for Products
const productSchema = new mongoose.Schema({
name: String,
price: Number,
description: String
});
const Product = mongoose.model('Product', productSchema);
// CRUD API Endpoints
// Fetch all products
app.get('/products', async (req, res) => {
try {
const products = await Product.find();
res.json(products);
} catch (error) {
res.status(500).send(error.message);
}
});
// Add a new product
app.post('/products', async (req, res) => {
try {
const newProduct = new Product(req.body);
await newProduct.save();
res.status(201).json(newProduct);
} catch (error) {
res.status(400).send(error.message);
}
});
// Update a product
app.put('/products/:id', async (req, res) => {
try {
const updatedProduct = await Product.findByIdAndUpdate(
req.params.id,
req.body,
{ new: true }
);
res.json(updatedProduct);
} catch (error) {
res.status(400).send(error.message);
}
});
// Delete a product
app.delete('/products/:id', async (req, res) => {
try {
await Product.findByIdAndDelete(req.params.id);
res.status(204).send();
} catch (error) {
res.status(500).send(error.message);
}
});
// Start Express server
const PORT = 4000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Step 6: Start the backend:
- Start the backend server using below command to see in the terminal message server is running on port 4000, connected to MongoDB.
node server.js
Step 6: Set up the Frontend:
- Open new terminal without deleting running backend server terminal.
- Execute 1st command to create frontend folder.
- After successfully creating product-client folder then change the directory using 2nd command.
npx create-react-app product-client
cd product-client
Step 7: Edit App.js and App.css file:
- Copy the below code and paste it into the App.js file and then save it.
- After then Copy the below code and paste it into the App.css file and then save it.
App.js:
import React, { useEffect, useState } from 'react';
import './App.css';
const App = () => {
const [products, setProducts] = useState([]);
const [newProduct, setNewProduct] = useState({
name: '',
price: '',
description: ''
});
const [editProduct, setEditProduct] = useState(null);
const [errors, setErrors] = useState({});
const fetchProducts = () => {
fetch('http://localhost:4000/products')
.then(response => response.json())
.then(data => setProducts(data));
};
useEffect(() => {
fetchProducts();
}, []);
const handleInputChange = (e) => {
const { name, value } = e.target;
if (editProduct) {
setEditProduct({ ...editProduct, [name]: value });
} else {
setNewProduct({ ...newProduct, [name]: value });
}
setErrors({ ...errors, [name]: '' });
};
const validateInputs = (product) => {
const newErrors = {};
if (!product.name) newErrors.name = 'Product name is required.';
if (!product.price) newErrors.price = 'Product price is required.';
if (!product.description) newErrors.description = 'Product description is required.';
setErrors(newErrors);
return Object.keys(newErrors).length === 0;
};
const addProduct = () => {
if (!validateInputs(newProduct)) return;
fetch('http://localhost:4000/products', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(newProduct)
})
.then(response => response.json())
.then(() => {
fetchProducts();
setNewProduct({ name: '', price: '', description: '' });
});
};
const updateProduct = () => {
if (!validateInputs(editProduct)) return;
fetch(`http://localhost:4000/products/${editProduct._id}`, {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(editProduct)
})
.then(response => response.json())
.then(() => {
fetchProducts();
setEditProduct(null);
});
};
const deleteProduct = (productId) => {
if (window.confirm('Are you sure you want to delete this product?')) {
fetch(`http://localhost:4000/products/${productId}`, {
method: 'DELETE'
})
.then(() => fetchProducts());
}
};
return (
<div className="container">
<h1 className="title">Product Management</h1>
{products.length === 0 ? (
<p className="no-products-message">No products available. Please add a new product.</p>
) : (
<table className="product-table">
<thead>
<tr>
<th>Name</th>
<th>Price</th>
<th>Description</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
{products.map(product => (
<tr key={product._id} className="product-row">
<td>{product.name}</td>
<td>₹{product.price}</td>
<td>{product.description}</td>
<td>
<div className="action-buttons">
<button onClick={() => setEditProduct(product)} className="edit-button">Edit</button>
<button onClick={() => deleteProduct(product._id)} className="delete-button">Delete</button>
</div>
</td>
</tr>
))}
</tbody>
</table>
)}
<div className="form-section">
<h2 className="form-title">{editProduct ? 'Edit Product' : 'Add New Product'}</h2>
<div className="input-group">
<div className="input-wrapper">
<input
type="text"
name="name"
value={editProduct ? editProduct.name : newProduct.name}
onChange={handleInputChange}
placeholder="Product Name"
className="input-field"
/>
{errors.name && <p className="error-message">{errors.name}</p>}
</div>
<div className="input-wrapper">
<input
type="number"
name="price"
value={editProduct ? editProduct.price : newProduct.price}
onChange={handleInputChange}
placeholder="Product Price ₹"
className="input-field"
/>
{errors.price && <p className="error-message">{errors.price}</p>}
</div>
<div className="input-wrapper">
<textarea
name="description"
value={editProduct ? editProduct.description : newProduct.description}
onChange={handleInputChange}
placeholder="Product Description"
className="input-field"
rows={3}
/>
{errors.description && <p className="error-message">{errors.description}</p>}
</div>
</div>
{editProduct ? (
<button onClick={updateProduct} className="update-button">Update Product</button>
) : (
<button onClick={addProduct} className="add-button">Add Product</button>
)}
</div>
</div>
);
};
export default App;
App.css:
.container {
max-width: 1000px;
margin: 0 auto;
padding: 20px;
font-family: Arial, sans-serif;
background-color: #ffffff;
box-shadow: 0 5px 15px rgba(0, 0, 0, 0.1);
border-radius: 8px;
}
.title {
border-radius: 7px;
color: #fff;
background: #000;
padding: 10px 0;
font-size: 1.6rem;
text-align: center;
margin: 0;
}
.product-table {
width: 100%;
border-collapse: collapse;
margin: 20px 0;
background-color: #f9f9f9;
box-shadow: 0 5px 15px rgba(0, 0, 0, 0.05);
border-radius: 8px;
overflow: hidden;
}
.product-table thead {
background-color: #007bff;
color: white;
text-align: left;
font-size: 1rem;
}
.product-table th,
.product-table td {
padding: 12px 15px;
border: 1px solid #ddd;
}
.product-table tr {
transition: background-color 0.2s ease-in-out;
}
td button {
display: block;
}
.product-table tbody tr:nth-child(even) {
background-color: #f9f9f9;
}
.input-group {
display: flex;
flex-direction: column;
gap: 10px;
}
.input-field {
padding: 10px;
border: 1px solid #ccc;
border-radius: 4px;
}
.add-button,
.edit-button,
.update-button {
padding: 10px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
transition: background-color 0.2s;
}
.add-button {
font-weight: 500;
margin-top: 10px;
background-color: #28a745;
color: white;
}
.add-button:hover {
background-color: #218838;
}
.edit-button {
background-color: #007bff;
color: white;
}
.edit-button:hover {
background-color: #0056b3;
}
.update-button {
margin-top: 20px;
background-color: #ffc107;
color: black;
font-weight: 600;
}
.update-button:hover {
background-color: #e0a800;
}
.action-buttons {
display: flex;
gap: 10px;
justify-content: center;
align-items: center;
}
.form-section {
margin-top: 40px;
}
.form-title {
width: fit-content;
background: #0000001a;
padding: 7px 7px;
border-radius: 7px;
font-size: 16px;
color: #000000;
}
.delete-button {
background-color: #dc3545;
color: white;
padding: 10px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
}
.delete-button:hover {
background-color: #c82333;
}
.no-products-message {
text-align: center;
font-size: 1.2rem;
color: #666;
margin-top: 20px;
padding: 10px;
background-color: #f9f9f9;
border-radius: 8px;
}
.error-message {
color: red;
background-color: #ffe6e6;
padding: 5px;
border-radius: 5px;
margin-top: 5px;
font-size: 0.9rem;
}
.input-wrapper {
display: flex;
flex-direction: column;
justify-content: center;
margin-bottom: 10px;
}
Step 8: Start the development server:
- Copy the below command to run the frontend to see the app.
npm start
OUTPUT:
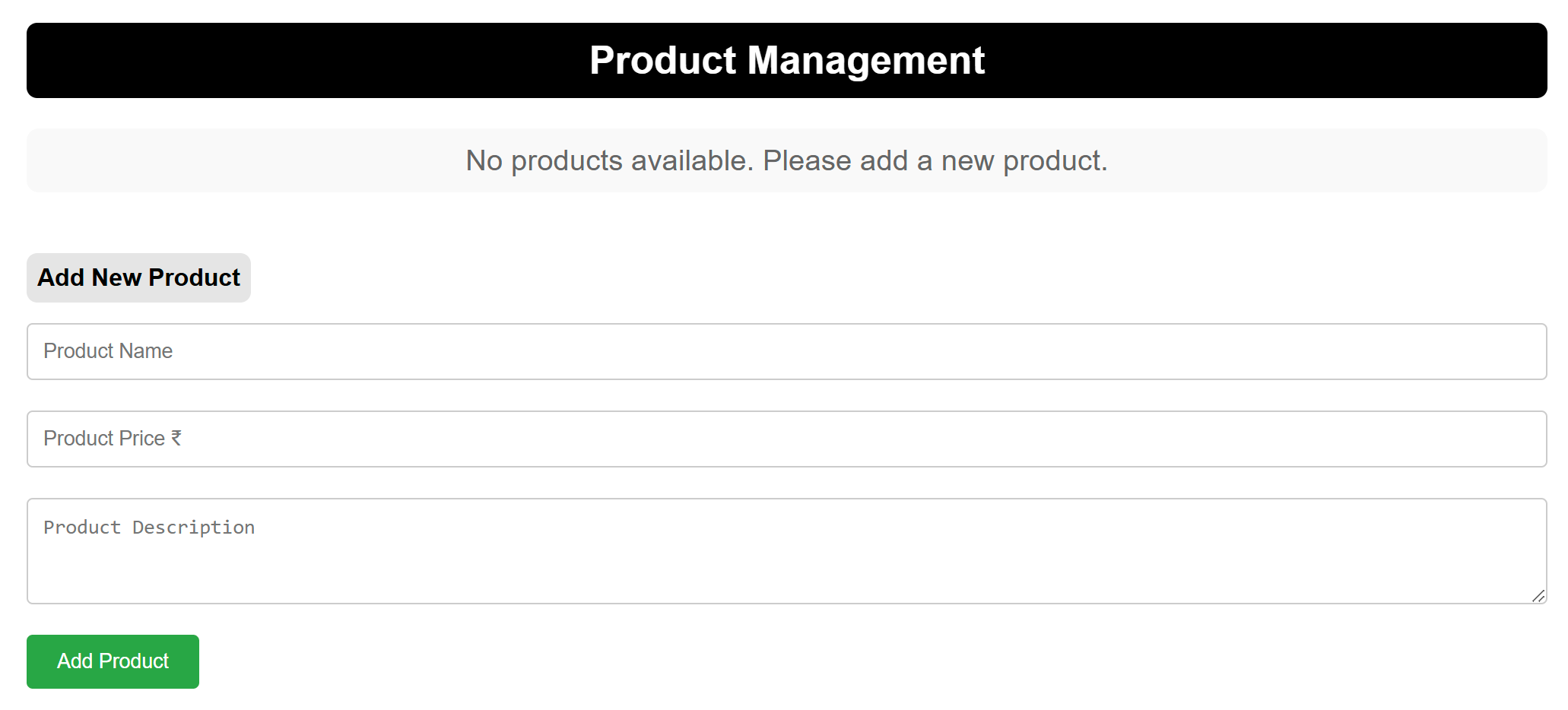
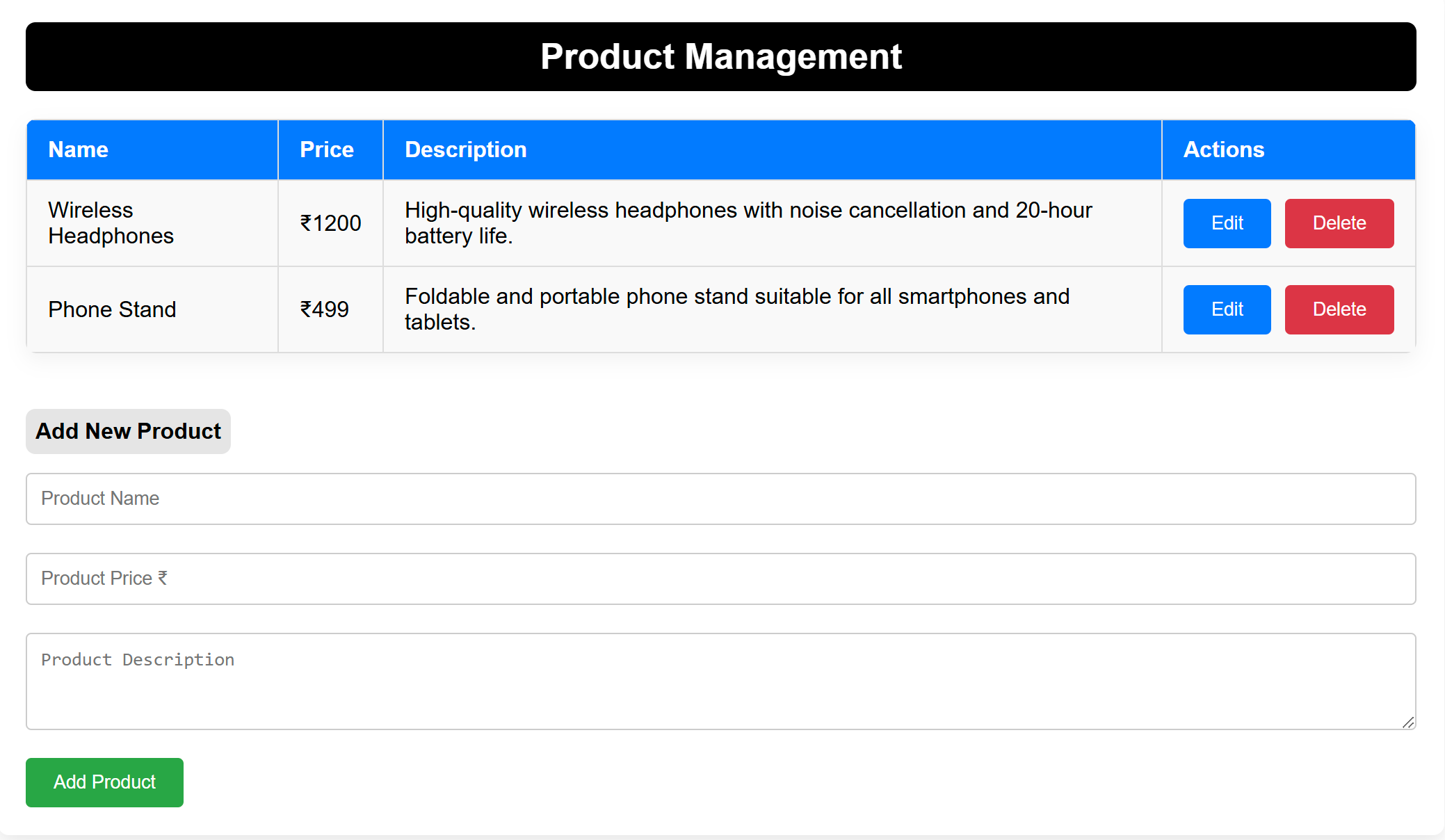
“Fixing the Module not found: Error: Can't resolve 'web-vitals'
Error in React”
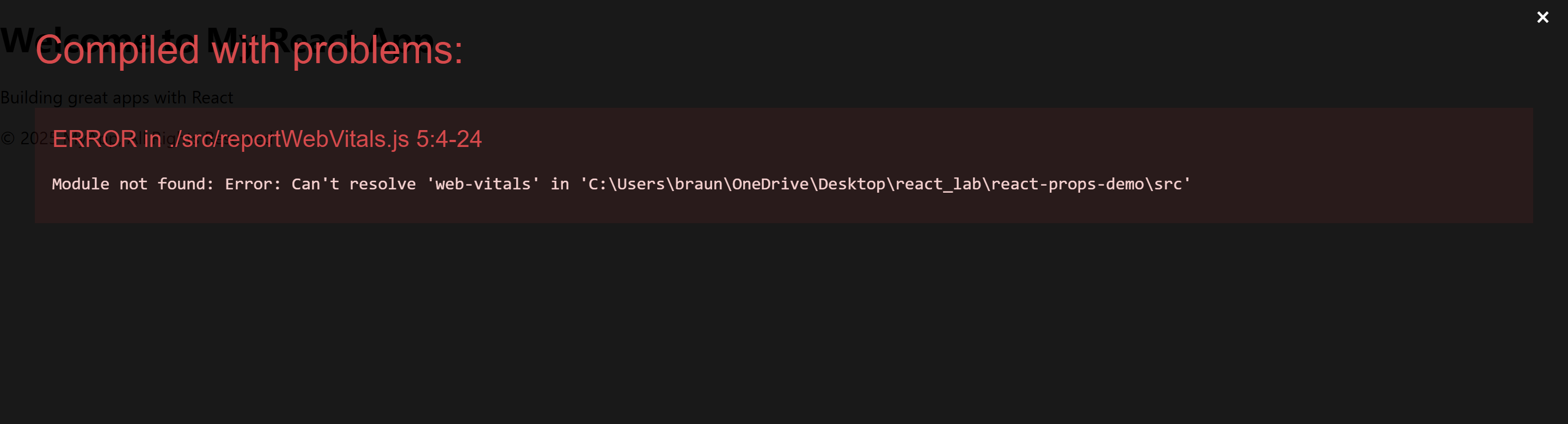
The error you’re seeing occurs because the web-vitals
package, which is used for performance monitoring in a React app, is not installed by default in the project or has been removed. Since web-vitals
is an optional package, you can safely resolve this issue by either installing the package or removing the code that imports it.
Option 1: Install the web-vitals
package
If you want to keep the performance monitoring functionality and resolve the error, simply install the web-vitals
package.
- In the terminal, navigate to your project folder (if not already there):
cd product-client
- Install
web-vitals
by running the following command:
npm install web-vitals
- After installation is complete, restart the development server:
npm start
This should resolve the error, and your application should compile correctly.
Option 2: Remove the Web Vitals Code (If Not Needed)
If you don’t need performance monitoring and want to get rid of the error, you can safely remove the import and usage of web-vitals
from your code.
- Open
src/reportWebVitals.js
and remove its contents or just comment out the code:
// import { reportWebVitals } from './reportWebVitals';
// You can safely remove the call to reportWebVitals or leave it commented out
// reportWebVitals();
- Save the file, and the application should compile without the error. You can now continue developing your app.
interesting program